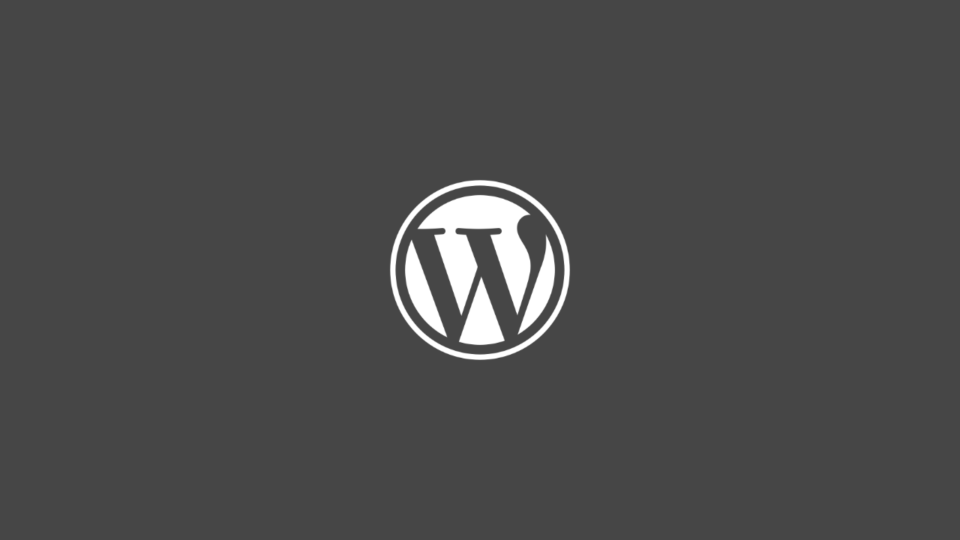
[WordPress] 特定のユーザーだけが編集できるカスタム投稿タイプ
WordPress で、カスタム投稿タイプを追加してページを作成し、そのページの編集のみを特定のユーザーに任せるという運用方法のウェブサイトを作ることがあったので、その備忘録を残します。
例えば企業サイトで、ページの作成・更新・削除は Web 部門が統括するけど、特定のページの編集は各部門に任せる、といったウェブサイトを作る際の参考になるかもしれません。
カスタム投稿タイプの追加
特定の権限グループが付与されたユーザーのみが操作できるカスタム投稿タイプを作るには、次のような PHP コードを functions.php に追加します。
add_action( 'init', function () {
// 新しい投稿タイプ
$post_type = 'new_post';
// 新しい投稿タイプの複数形
$plural_post_type = 'new_posts';
// 新しい投稿タイプの独自権限
$capabilities = [
'delete_post' => "delete_{$post_type}",
'edit_post' => "edit_{$post_type}",
'read_post' => "read_{$post_type}",
'delete_posts' => "delete_{$plural_post_type}",
'delete_others_posts' => "delete_others_{$plural_post_type}",
'delete_private_posts' => "delete_private_{$plural_post_type}",
'delete_published_posts' => "delete_published_{$plural_post_type}",
'edit_posts' => "edit_{$plural_post_type}",
'edit_others_posts' => "edit_others_{$plural_post_type}",
'edit_private_posts' => "edit_private_{$plural_post_type}",
'edit_published_posts' => "edit_published_{$plural_post_type}",
'publish_posts' => "publish_{$plural_post_type}",
'read_private_posts' => "read_private_{$plural_post_type}",
];
// 新しい投稿タイプの登録
register_post_type( $post_type, [
…
'capability_type' => [ $post_type, $plural_post_type ],
'capabilities' => $capabilities,
'map_meta_cap' => true,
…
] );
// 管理者に新しい投稿タイプの権限を追加
$administrator = get_role( 'administrator' );
foreach ( $capabilities as $capability ) {
$administrator->add_cap( $capability );
}
} );
カスタム投稿タイプを追加した場合、通常は「post (投稿)」や「page (固定ページ)」と同じ権限グループで操作ができます。
今回は、追加した「new_post (新しい投稿タイプ)」の編集だけができる権限グループを作るので、独自権限を作る必要があります。
独自権限を作るには、register_post_type
の第二引数の「capability_type
」「capabilities
」「map_meta_cap
」を設定します。
ちなみに、register_post_type
の引数に関しては「register_post_type()」、権限一覧は「Roles and Capabilities – WordPress.org Documentation」で確認できます。
独自権限なので、権限グループの「管理者」も操作できるよう、権限を追加しておくことも忘れずに。
権限グループの追加
権限グループには、デフォルトで「管理者」「編集者」「投稿者」「寄稿者」「購読者」の5つがあります。これに、独自の権限グループ「専任者」を追加するには、次のような PHP コードを functions.php に追加します。
add_action( 'init', function () {
// 新しい投稿タイプの複数形
$plural_post_type = 'new_posts';
// ロールオブジェクトを取得
$roles = wp_roles();
// 新しい権限グループに新しい投稿タイプの権限を追加
$role = 'new_role';
$roles->add_role( $role, '専任者' );
$roles->add_cap( $role, "edit_{$plural_post_type}" );
$roles->add_cap( $role, "edit_published_{$plural_post_type}" );
$roles->add_cap( $role, 'upload_files' );
$roles->add_cap( $role, 'read' );
} );
この設定により、「専任者」のユーザーは「new_post (新しい投稿タイプ)」のページ編集のみができるようになります。ページの作成・更新・削除は「管理者」が行います。
作成したページを「専任者」のユーザーが編集できるよう、ページの「投稿者」をそのユーザーに変更すれば完了です。イメージとしては、編集だけができる「寄稿者」のようになります。
まとめ
「カスタム投稿タイプの追加」と「権限グループの追加」の PHP コードをひとまとめにすると、次のようになります。
add_action( 'init', function () {
// 新しい投稿タイプ
$post_type = 'new_post';
// 新しい投稿タイプの複数形
$plural_post_type = 'new_posts';
// 新しい投稿タイプの独自権限
$capabilities = [
'delete_post' => "delete_{$post_type}",
'edit_post' => "edit_{$post_type}",
'read_post' => "read_{$post_type}",
'delete_posts' => "delete_{$plural_post_type}",
'delete_others_posts' => "delete_others_{$plural_post_type}",
'delete_private_posts' => "delete_private_{$plural_post_type}",
'delete_published_posts' => "delete_published_{$plural_post_type}",
'edit_posts' => "edit_{$plural_post_type}",
'edit_others_posts' => "edit_others_{$plural_post_type}",
'edit_private_posts' => "edit_private_{$plural_post_type}",
'edit_published_posts' => "edit_published_{$plural_post_type}",
'publish_posts' => "publish_{$plural_post_type}",
'read_private_posts' => "read_private_{$plural_post_type}",
];
// 新しい投稿タイプの登録
register_post_type( $post_type, [
...
'capability_type' => [ $post_type, $plural_post_type ],
'capabilities' => $capabilities,
'map_meta_cap' => true,
...
] );
// 管理者に新しい投稿タイプの権限を追加
$administrator = get_role( 'administrator' );
foreach ( $capabilities as $capability ) {
$administrator->add_cap( $capability );
}
// ロールオブジェクトを取得
$roles = wp_roles();
// 新しい権限グループに新しい投稿タイプの権限を追加
$role = 'new_role';
$roles->add_role( $role, '専任者' );
$roles->add_cap( $role, "edit_{$plural_post_type}" );
$roles->add_cap( $role, "edit_published_{$plural_post_type}" );
$roles->add_cap( $role, 'upload_files' );
$roles->add_cap( $role, 'read' );
} );
〆
暑い。